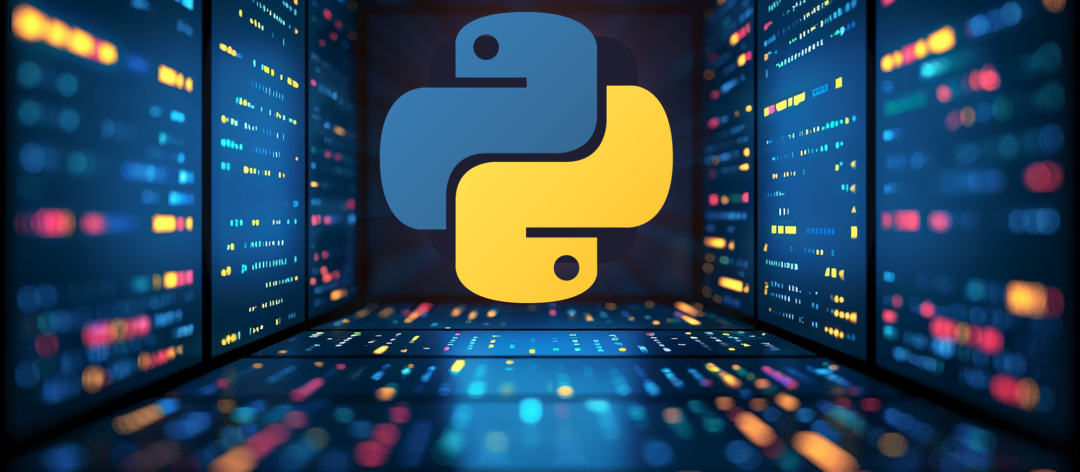
Python是一种多才多艺、被广泛使用的编程语言,拥有大量的库和框架。总结了近60个常用或较少人知晓的Python技巧的代码片段,每一个都能够为您的编程工具箱增添新的维度。从优化数据结构到简化算法,提高可读性到提升性能,这些代码片段不仅仅是代码 —— 它们是解锁Python全部潜力的关键。
上一篇结合30个代码技巧片段 《提升python编程技能的60个代码片段(上)》,接下来继续另外30个。
31.next with default
从迭代器中获取下一个项,如果用尽则返回默认值。
it = iter([1, 2, 3])
print(next(it, 'done'))
print(next(it, 'done'))
print(next(it, 'done'))
print(next(it, 'done'))
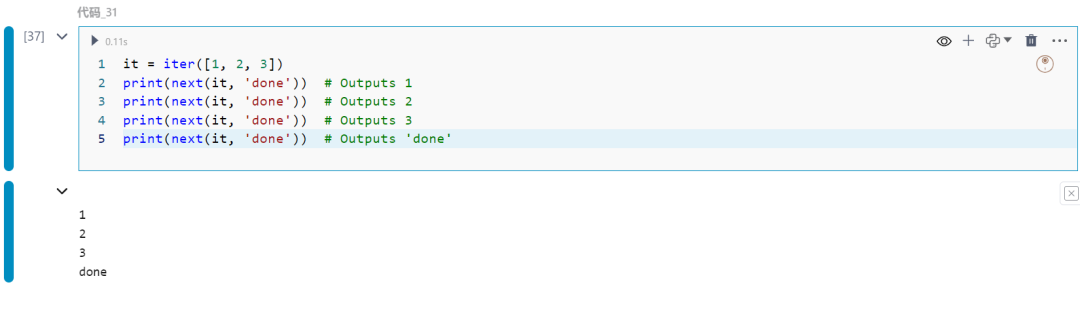
32.operator.itemgetter
高效地从集合中获取项目。
from operator import itemgetter
data = [('Alice', 25), ('Bob', 30), ('Charlie', 35)]
print(sorted(data, key=itemgetter(1)))
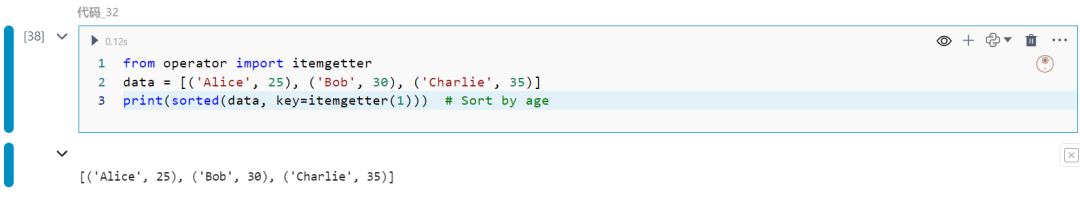
33.operator.attrgetter
从对象中获取属性。
from operator import attrgetter
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
people = [Person('Alice', 30), Person('Bob', 25)]
print(sorted(people, key=attrgetter('age')))
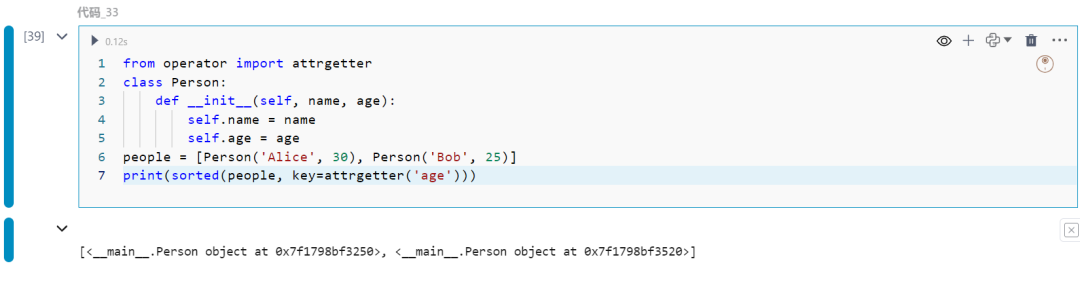
34.string.ascii_letters及相关常量
预定义的字符串常量。
import string
print(string.ascii_letters)
print(string.digits)
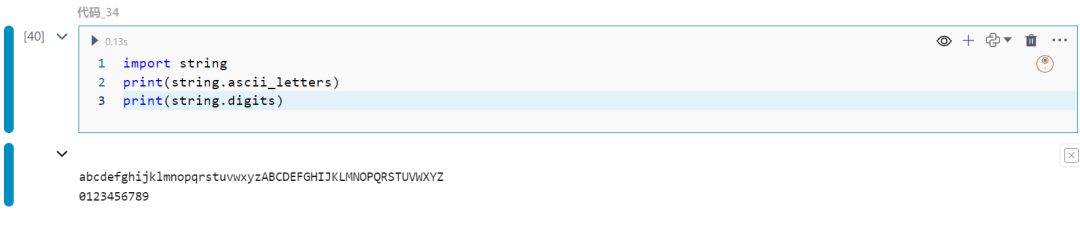
35.os.walk
遍历目录树。
import os
for root, dirs, files in os.walk('.'):
print(root, dirs, files)
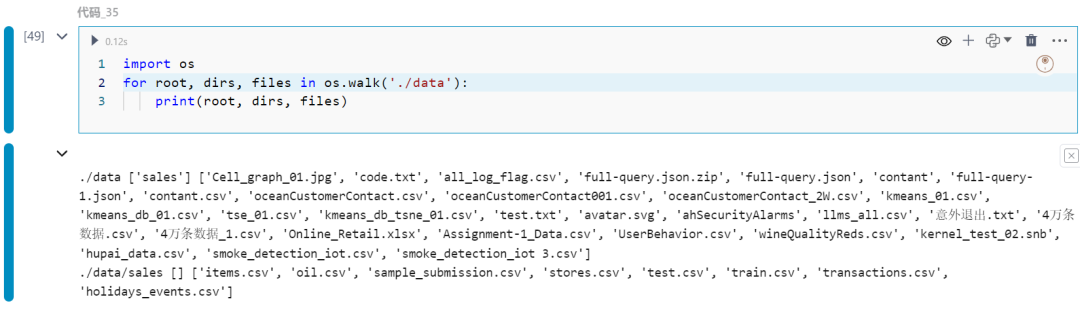
36.glob.glob
文件名的模式匹配。
import glob
for filename in glob.glob('*.txt'):
print(filename)
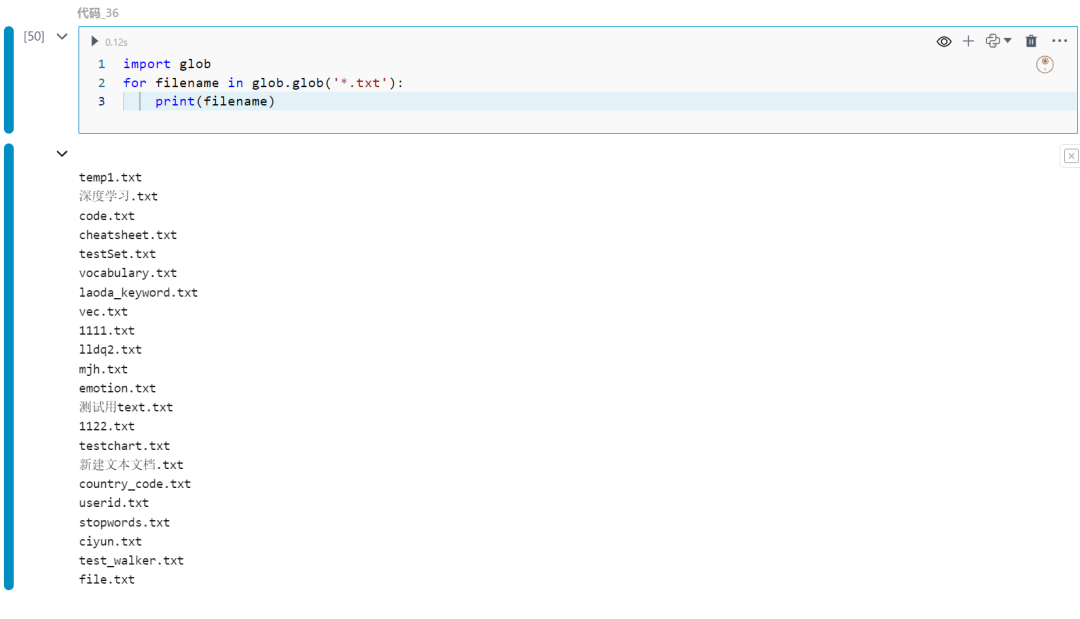
37.heapq模块
提供实现堆的函数。
import heapq
heap = [1, 3, 5, 7, 9, 2, 4, 6, 8, 0]
heapq.heapify(heap)
heapq.heappush(heap, -5)
print([heapq.heappop(heap) for _ in range(3)])
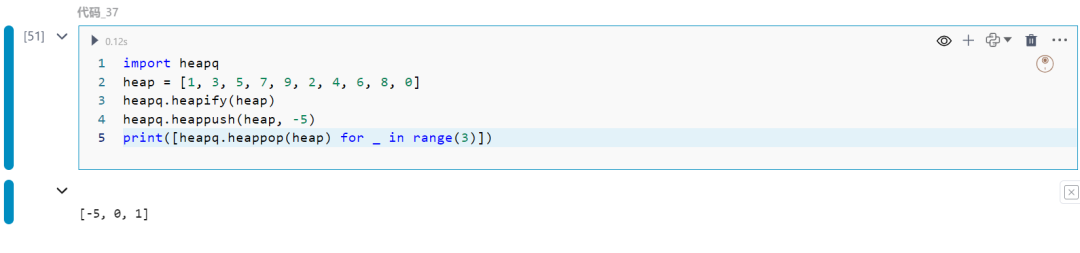
38.用于二分搜索的bisect模块
按排序顺序插入列表。
import bisect
a = [1, 2, 4, 5]
bisect.insort(a, 3)
print(a)
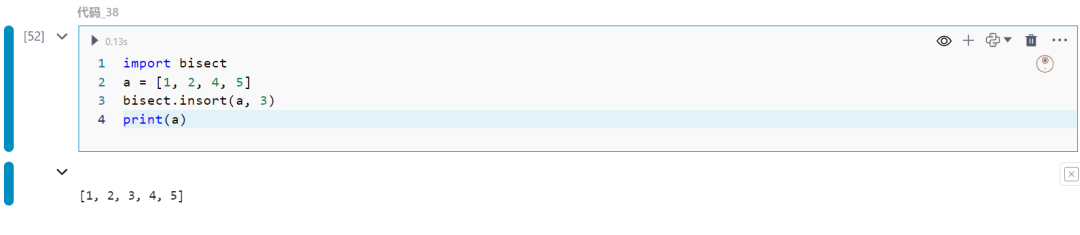
39.collections.deque
双端队列。
from collections import deque
d = deque('ghi')
d.append('j')
d.appendleft('f')
print(d)
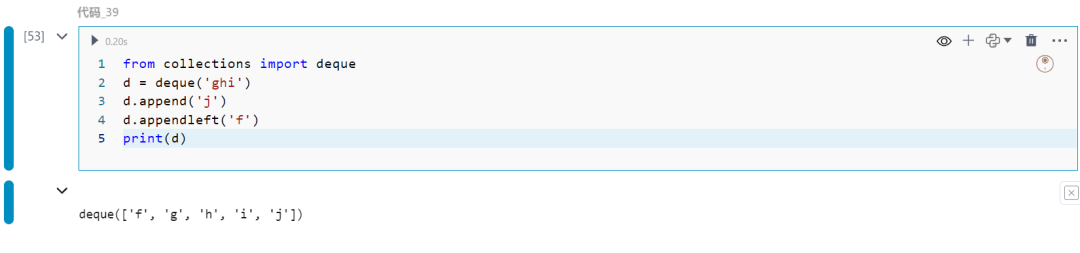
40.用于处理CSV文件的csv模块
读取和写入CSV文件。
import csv
with open('example.csv', 'w', newline='') as f:
writer = csv.writer(f)
writer.writerow(['Name', 'Age'])
writer.writerow(['Alice', 30])
writer.writerow(['Bob', 25])
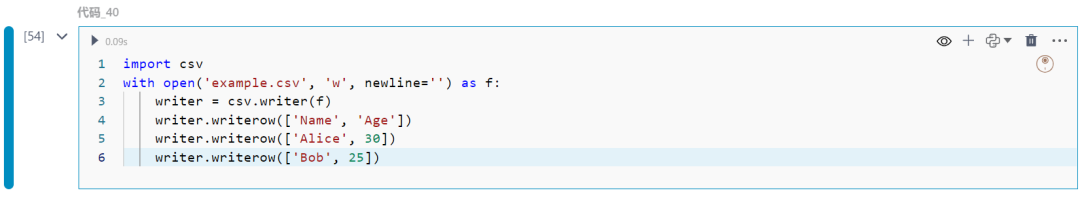
41.filter函数
根据函数过滤可迭代对象的元素。
numbers = [1, 2, 3, 4, 5]
even_numbers = filter(lambda x: x % 2 == 0, numbers)
print(list(even_numbers))
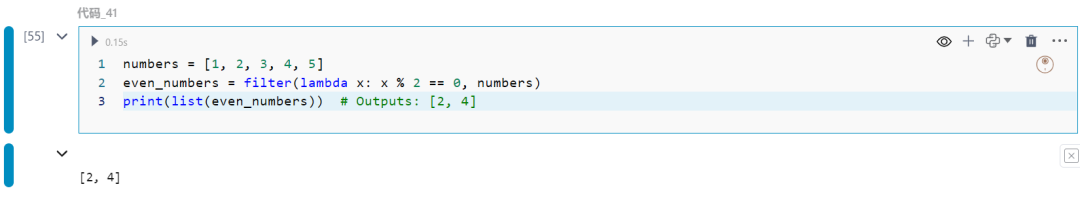
42.map函数
对可迭代对象的每个项应用函数。
squares = map(lambda x: x**2, numbers)
print(list(squares))

43.复数complex
创建复数。
c = complex(2, 3)
print(c)

44.类中的__dict__
访问对象的属性。
class MyClass:
def __init__(self):
self.a = 1
self.b = 2
obj = MyClass()
print(obj.__dict__)
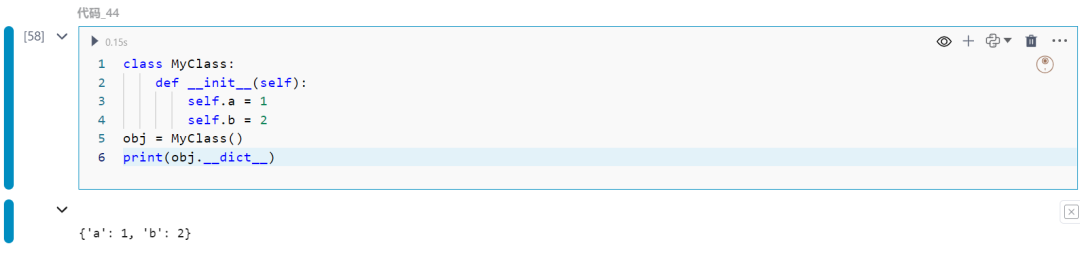
45.itertools.tee
从单个可迭代对象创建n个独立的迭代器。
import itertools
iter1, iter2 = itertools.tee(numbers, 2)
print(list(iter1), list(iter2))
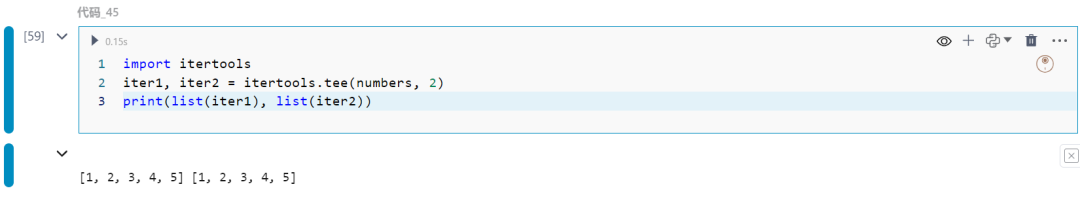
46.itertools.islice
迭代器的切片。
slice_iter = itertools.islice(range(10), 2, 6)
print(list(slice_iter))

47.itertools.starmap
类似于map,但参数来自可迭代对象。
data = [(2, 5), (3, 2), (10, 3)]
result = itertools.starmap(pow, data)
print(list(result))
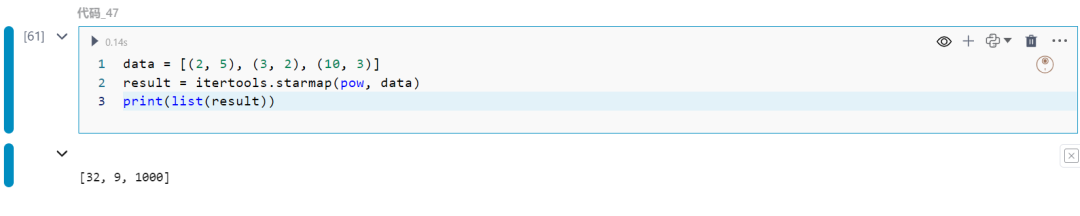
48.collections.ChainMap
将多个字典组合成单个视图。
from collections import ChainMap
dict1 = {'a': 1, 'b'
: 2}
dict2 = {'c': 3, 'd': 4}
combined = ChainMap(dict1, dict2)
print(combined['a'])
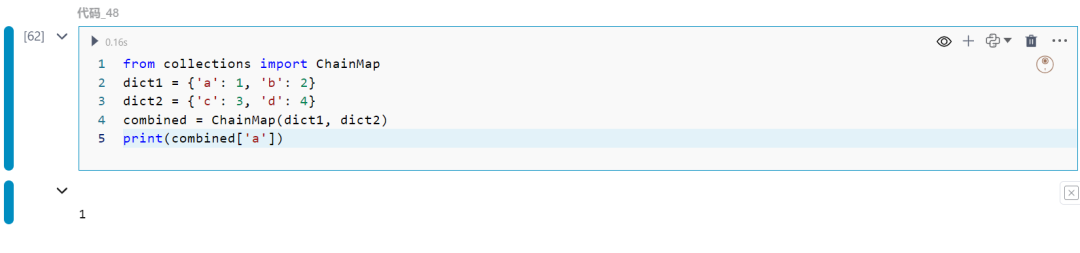
49.functools.reduce
对可迭代对象的项累加应用函数。
from functools import reduce
sum_of_numbers = reduce(lambda x, y: x + y, numbers)
print(sum_of_numbers)
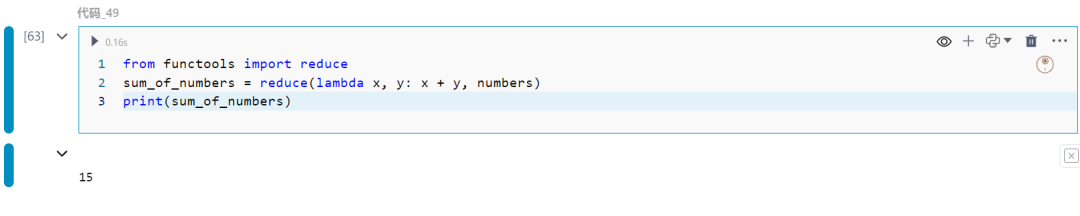
50.itertools.accumulate
返回累积和或其他二元函数。
import itertools
acc = itertools.accumulate(numbers, lambda x, y: x + y)
print(list(acc))
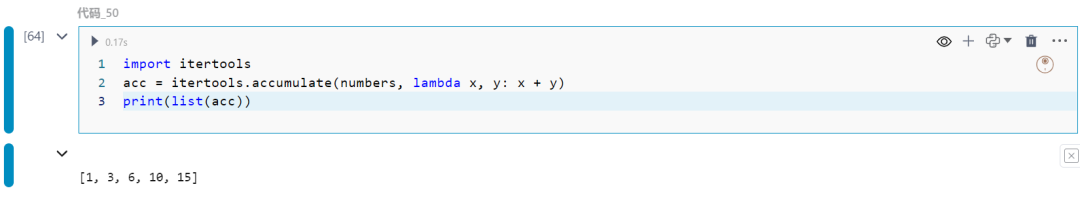
51.functools.total_ordering
用于自动生成排序方法的装饰器。
from functools import total_ordering
@total_ordering
class MyClass:
def __init__(self, value):
self.value = value
def __eq__(self, other):
return self.value == other.value
def __lt__(self, other):
return self.value < other.value
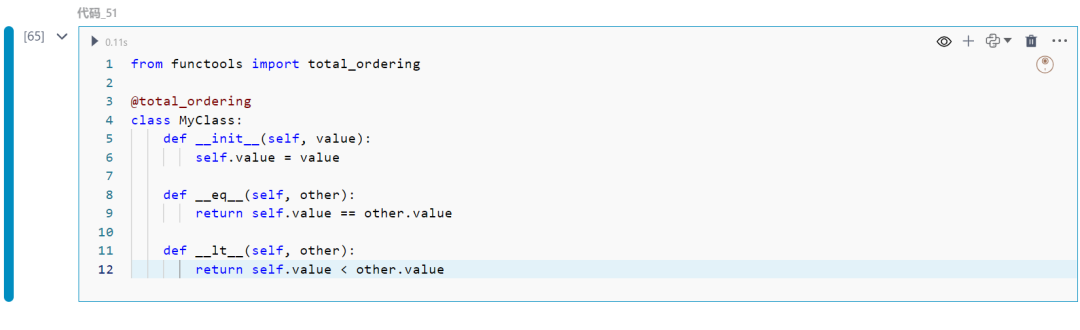
52.hashlib用于散列
从字符串生成散列值。
import hashlib
hash_value = hashlib.sha256(b'Hello World').hexdigest()
print(hash_value)
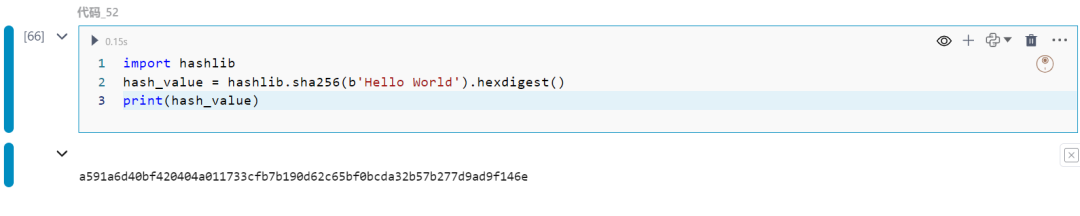
53.sys.getsizeof
获取对象的字节大小。
import sys
num = 5
print(sys.getsizeof(num)) # Outputs: Size in bytes
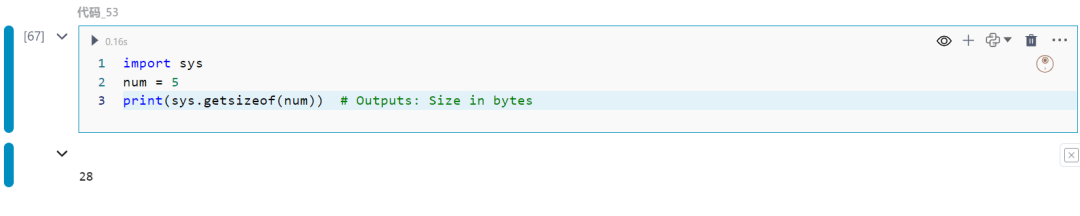
54.shlex.split
使用类似shell的语法拆分字符串。
import shlex
cmd = 'ls -l /var/www'
print(shlex.split(cmd))
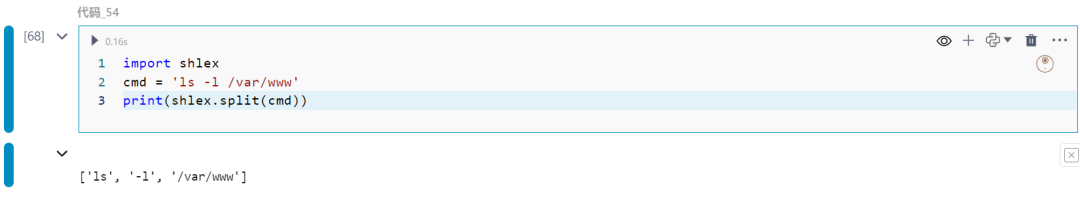
55.os.environ
访问环境变量。
import os
print(os.environ['PATH'])

56.日历模块
与日历相关的有用函数。
import calendar
print(calendar.isleap(2024))

57.uuid模块
用于生成唯一标识符。
import uuid
unique_id = uuid.uuid4()
print(unique_id)
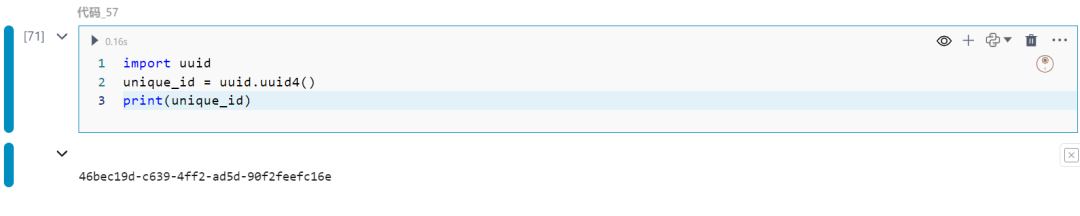
58.异步编程的asyncio模块
使用async/await语法编写并发代码。
import asyncio
async def main():
await asyncio.sleep(1)
print('Hello')
asyncio.run(main())
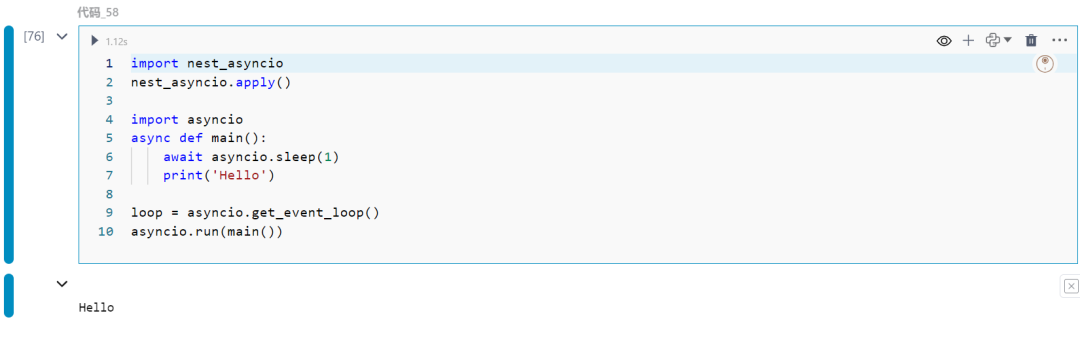
59.name == "main"
检查脚本是否直接运行。
if __name__ == "__main__":
print("Script run directly")

60.用于漂亮打印的pprint模块
以格式化的方式打印Python数据结构。
import pprint
data = {'a': [1, 2, 3], 'b': [4, 5, 6]}
pprint.pprint(data)
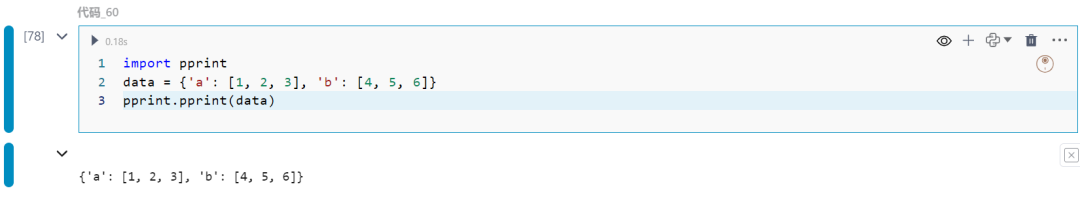
上述探索的30个Python隐藏功能和函数,和上一篇《提升python编程技能的60个代码片段(上)》一起揭示了Python的深度和多样性。从使用较少人知晓的数据结构和itertools增强代码效率,通过上下文管理器和装饰器确保强大且更清洁的代码,到利用面向对象特性和内存管理的全部潜力,这些元素突显了Python满足各种编程需求的能力。