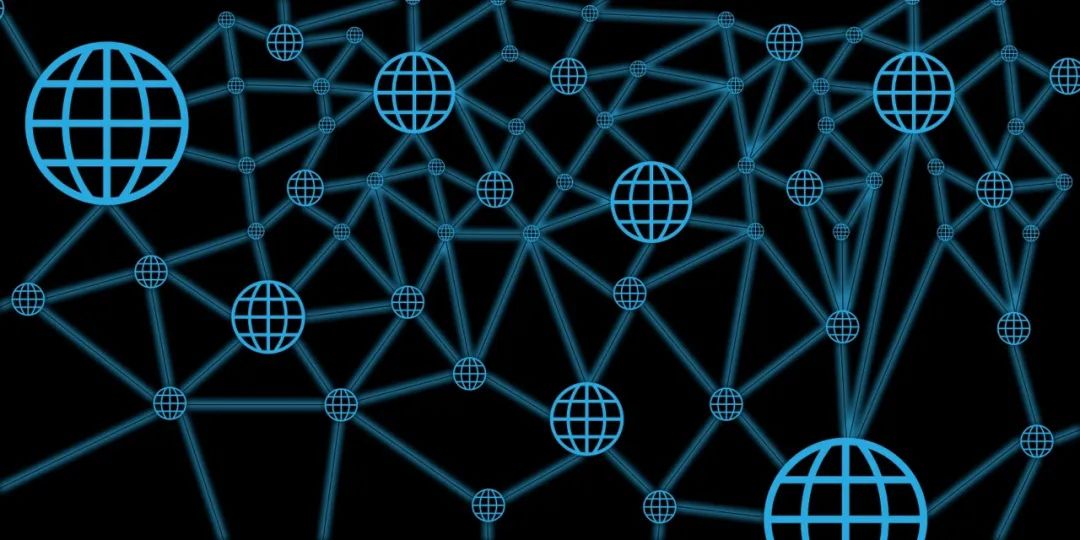
本文主要介绍如何在Python中使用The Graph来查询以太坊数据。The Graph项目是一个用于查询去中心化网络的索引系统。你可以使用The Graph来查询Ethereum、IPFS等系统。
在我们开始之前,让我们先来看看一些定义。
Graph 项目使用 GraphQL,这是一种描述如何询问数据的语法。这种语法并不与特定类型的数据库或存储引擎挂钩,而是以你现有的代码和数据为支撑。
GraphQL
让我们先看看一个非常简单的GraphQL查询结构,以及我们运行它时得到的结果。一旦GraphQL服务开始运行,它就可以接收GraphQL查询语句来执行。该服务检查查询语句,以确保它只关联定义的类型和字段,然后运行函数以产生结果。
作为一个例子,查看下面的查询结构:
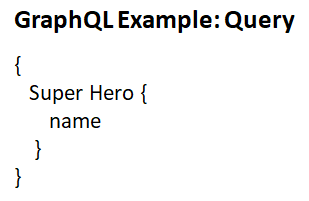
上面的GraphQL查询可以产生以下结果:
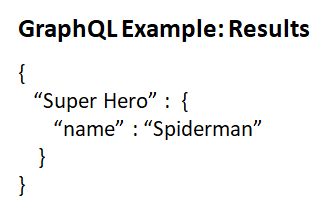
你可以看到,查询的结构与结果相同。这对GraphQL至关重要,因为服务器可以确切地知道客户要求的是什么字段。
我们可以使用GraphQL来进行以下操作:
可以访问GraphQL官网,了解更多关于如何编写复杂的GraphQL查询。
https://graphql.org/learn/queries/
The Graph
为了更好地了解The Graph项目是什么以及它如何工作,请访问 thegraph.com/docs
。它解释了如何部署一个 subgraph
以及如何查询 subgraph
的数据。一个 subgraph
定义了 TheGraph
将从以太坊索引哪些数据,以及如何存储这些数据。一旦 subgraph
被部署,就可以使用GraphQL语法进行查询。
在本教程中,我们将专注于从 subgraph
中查询数据。
1、访问The Graph Explorer( https://thegraph.com/explorer/
),查看以太坊区块链存在的所有托管subgraph。这些托管服务(subgraphs)中的每一个都可以被查询到数据。
2、选择一个 subgraphs
页面,并注意该页面的http查询地址和 Playground
。
3、在你的Python代码中需要http查询地址,它是包含区块链数据的端点。这个服务将执行你的GraphQL查询。
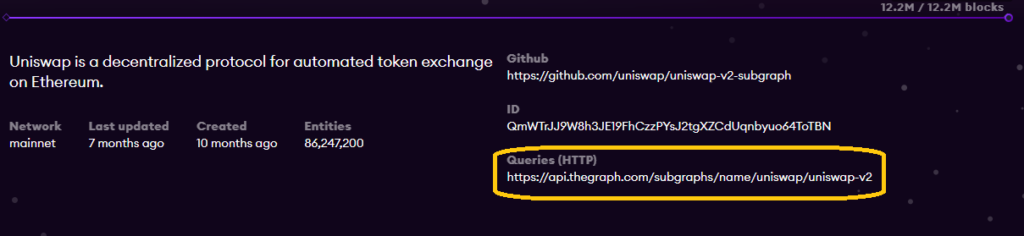
4.确保你在 Playground
上进行实验。该网站的这一部分将允许你构建和测试你的GraphQL Ethereum区块链查询。
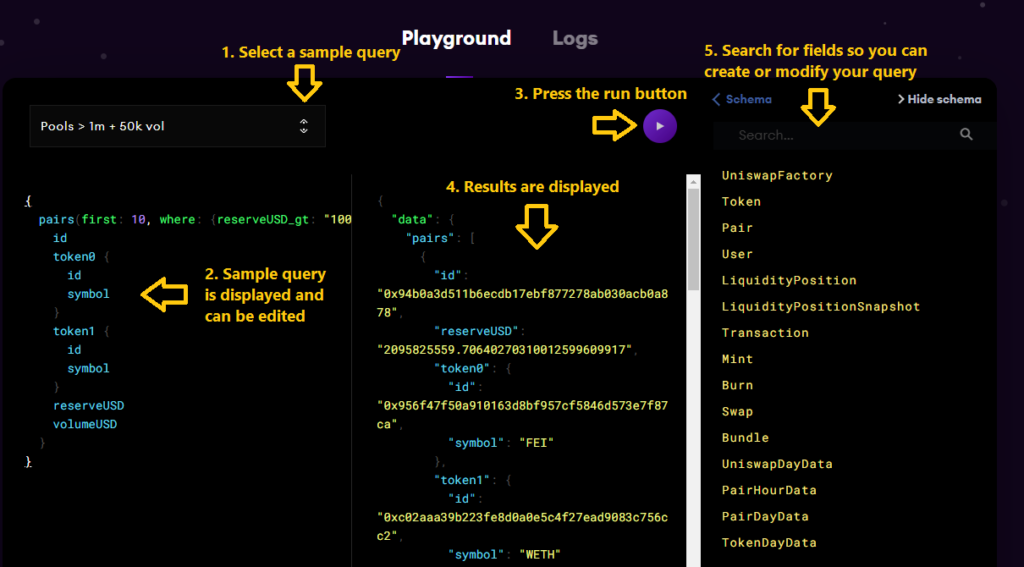
在Python中使用The Graph
接下来基于我们在The Graph的Playground中构建的一些查询,可以在我们的Python代码中使用它来请求来自Ethereum区块链的不同数据。
下面的Python示例代码包含一个通用函数,用于向一个 subgraph
发出帖子请求。为了使用不同的
subgraph
,你需要改变url端点和GraphQL语法。我在程序的末尾包含了一个打印语句(更容易阅读),所以来自Ethereum区块链的结果会在你的控制台中打印出来。
例1:使用Python中的GraphQL查询以太坊区块链上的Aave,以获得按时间戳划分的最近10笔闪电贷款的列表
import requests
# pretty print is used to print the output in the console in an easy to read format
from pprint import pprint
# function to use requests.post to make an API call to the subgraph url
def run_query(q):
# endpoint where you are making the request
request = requests
.post('https://api.thegraph.com/subgraphs/name/aave/protocol'
'',
json={'query': query})
if request.status_code == 200:
return request.json()
else:
raise Exception(
'Query failed. return code is {}. {}'.format(request.status_code, query))
# The Graph query - Query aave for a list of the last 10 flash loans by time stamp
query = """
{
flashLoans (first: 10, orderBy: timestamp, orderDirection: desc,){
id
reserve {
name
symbol
}
amount
timestamp
}
}
"""
result = run_query(query)
# print the results
print('Print Result - {}'.format(result
))
print('#############')
# pretty print the results to make it easier to read
pprint(result)
例2:使用Python中的GraphQL查询以太坊区块链上的Uniswap,以获得前10对的列表
下面的查询是Uniswap的一个排行榜,详细介绍了按ETH存入量降序排列的顶级ETH流动性供应商。这可以帮助你更好地分析用户行为,比如跟踪市场上的热门人物,观察ETH的流动性供应商与其他代币之间的关系。其他可以查询的用户字段包括他们的地址,历史购买和出售的资产以及该用户支付的总费用。
import requests
# pretty print is used to print the output in the console in an easy to read format
from pprint import pprint
# function to use requests.post to make an API call to the subgraph url
def run_query(q):
# endpoint where you are making the request
request = requests.post('https://api.thegraph.com/subgraphs/name/uniswap/uniswap-v2'
'',
json={'query': query})
if request.status_code == 200:
return request.json()
else:
raise Exception('Query failed. return code is {}. {}'.format(request.status_code, query))
# The Graph query - Query Uniswap for a list of the top 10 pairs where the reserve is > 1000000 USD and the volume is >50000 USD
query = """
{
pairs(first: 10, where: {reserveUSD_gt: "1000000", volumeUSD_gt: "50000"}, orderBy: reserveUSD, orderDirection: desc) {
id
token0 {
id
symbol
}
token1 {
id
symbol
}
reserveUSD
volumeUSD
}
}
"""
result = run_query(query)
# print the results
print('Print Result - {}'.format(result))
print('#############')
# pretty print the results
pprint(result)
在Python中使用The Graph来查询Ethereum数据是非常强大的。有很多数据可以被查询,用于生成报告和分析。
此代码仅用于学习和娱乐目的。该代码没有经过审计,使用风险自负,合约是实验性质的,可能包含bug。